I implemented to code to check for a collision with a block and it is super tricky and I know I got it wrong but I need help figuring out what is correct.Before this segment of code, the blocks formed just fine in 8 columns and 4 rows. I have included a screenshot of what they look like after this segment, and the red box shows which blocks do what I actually want them to. all other blocks just sit there.
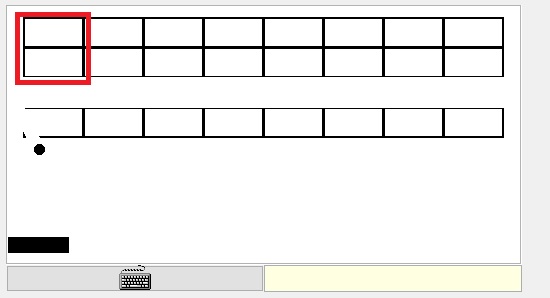
Here is the code I have:
//if the ball collides with a block
let temp = 0;
while (temp < numBlocks)
{
let tempBlock = blockArray[temp];
let blockX = tempBlock.getX();
let blockY = tempBlock.getY();
let blockSize = tempBlock.getSize();
if (tempBlock.show())
{
if (((ballY + ballRad) > blockY) & ((ballY - ballRad) < (blockY + (blockSize/2))))
{
if (((ballX + ballRad) = blockX) | ((ballX - ballRad) < (blockX + blockSize)))
{
do tempBlock.erase();
let vert = ~vert;
let numBlocks = numBlocks - 1;
}
}
}
let temp = temp + 1;
if (numBlocks = 0)
{
let lose = true;
}
}