Thanks for the help! I think I have been pretty good about doing incremental changes to my work, and testing each step of the way. The challenging bugs though are the ones that don't even through any errors, and just output something unexpected. here is an example:
I have written and tested each of the libraries in the OS using the tests that I got in the project 12 folder. As one final test I tried to run the Pong game that was shown in project 11 using my OS, and it seemed to be mostly working however there are a couple bugs. I have ruled out my compiler as the issue.
One bug is that the paddle leaves an after image, and has white lines running through it. Here is the second frame of the pong game running using my OS:
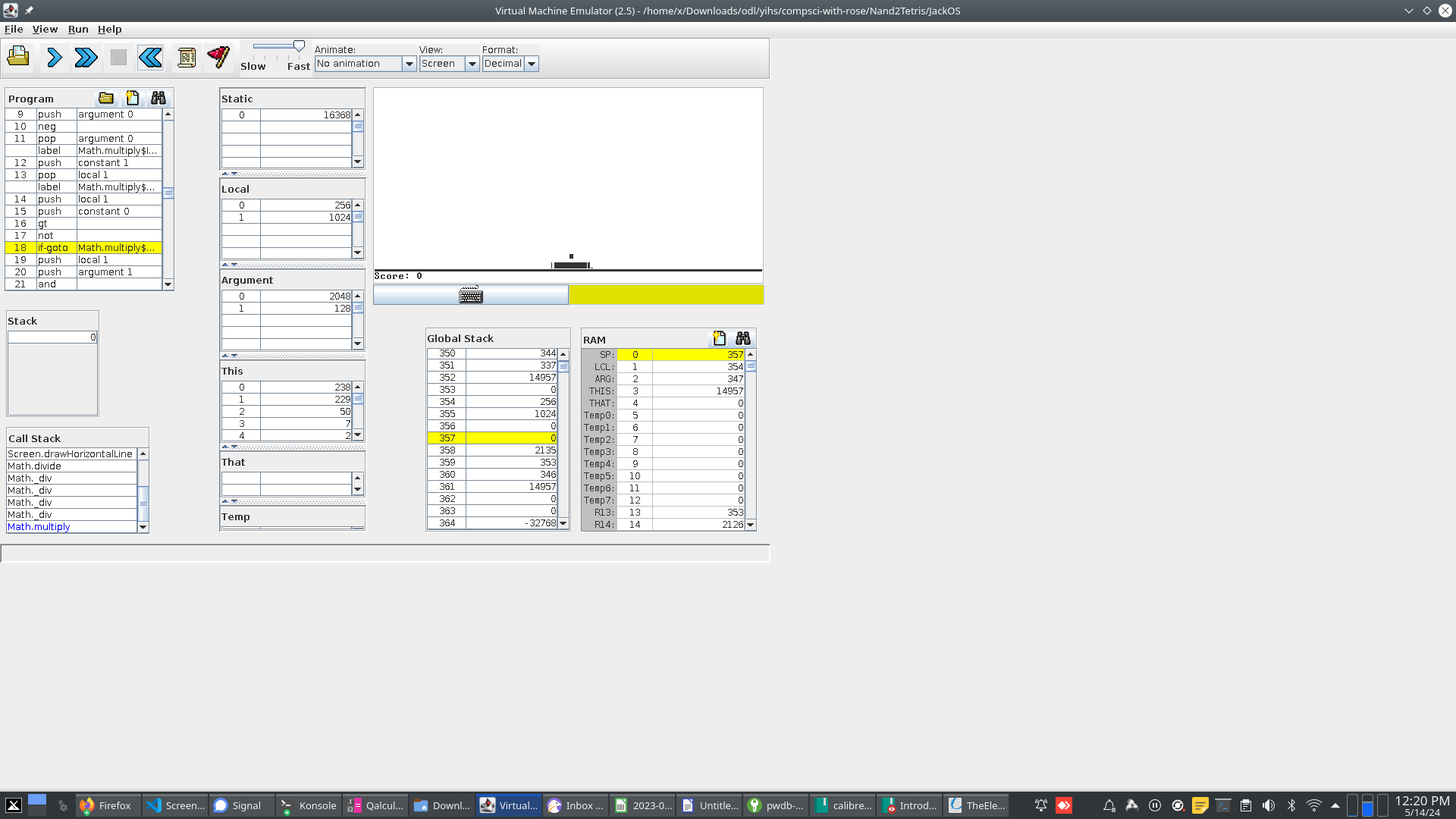
The paddle is moving right, and as you can see, it has left behind a black line and acquired a white one inside of it. Here is the code for my screen library where I would guess the bug is occurring:
class Screen {
static boolean color;
static int newBit;
static Array powers_of_two, screenMem;
function void init() {
let screenMem = 16384;
let color = true;
let powers_of_two = Array.new(16);
let powers_of_two[0] = 1;
let powers_of_two[1] = 2;
let powers_of_two[2] = 4;
let powers_of_two[3] = 8;
let powers_of_two[4] = 16;
let powers_of_two[5] = 32;
let powers_of_two[6] = 64;
let powers_of_two[7] = 128;
let powers_of_two[8] = 256;
let powers_of_two[9] = 512;
let powers_of_two[10] = 1024;
let powers_of_two[11] = 2048;
let powers_of_two[12] = 4096;
let powers_of_two[13] = 8192;
let powers_of_two[14] = 16384;
let powers_of_two[15] = 16384+16384;
return;
}
function void clearScreen() {
var int screenCell;
let screenCell = 8192;
while (screenCell) {
let screenCell = screenCell - 1;
let screenMem[screenCell] = 0;
}
return;
}
function void setColor(boolean newColor) {
let color = newColor;
return;
}
function void drawPixel(int x, int y) {
var int address, pixelBit;
let address = (y * 32) + (x / 16);
let pixelBit = powers_of_two[x & 15];
if (color) {let screenMem[address] = screenMem[address] | pixelBit;}
else {let screenMem[address] = screenMem[address] & ~pixelBit;}
return;
}
function void drawLine(int x1, int y1, int x2, int y2) {
var int dx, dy, a, b, diff, temp, address, pixelBit;
if (x1 > x2) {
let temp = x1;
let x1 = x2;
let x2 = temp;
let temp = y1;
let y1 = y2;
let y2 = temp;
}
let dx = x2 - x1;
let dy = y2 - y1;
if (dx = 0) {
if (y1 > y2) {
let temp = y1;
let y1 = y2;
let y2 = temp;
}
let address = (y1 * 32) + (x1 / 16);
let pixelBit = powers_of_two[x1 & 15];
let y2 = y2 + 1;
while (y1 < y2) {
if (color) {let screenMem[address] = screenMem[address] | pixelBit;}
else {let screenMem[address] = screenMem[address] & ~pixelBit;}
let address = address + 32;
let y1 = y1 + 1;
}
} else { if (dy = 0) {
do Screen.drawHorizontalLine(x1, x2, y1);
} else {
if (y1 < y2) {
while (~(a > dx) & ~(b > dy)) {
do Screen.drawPixel(x1 + a, y1 + b);
if (diff < 0) {let a = a + 1; let diff = diff + dy;}
else {let b = b + 1; let diff = diff - dx;}
}
} else {
while (~(a > dx) & ~(b < dy)) {
do Screen.drawPixel(x1 + a, y1 + b);
if (diff < 0) {let a = a + 1; let diff = diff - dy;}
else {let b = b - 1; let diff = diff - dx;}
}
}
}}
return;
}
function void drawRectangle(int x1, int y1, int x2, int y2) {
var int dx, dy, temp;
if (x1 > x2) {
let temp = x2;
let x2 = x1;
let x1 = temp;
}
if (y1 > y2) {
let temp = y2;
let y2 = y1;
let y1 = temp;
}
let dx = x2 - x1;
let dy = y2 - y1;
while (dy > 0) {
do Screen.drawHorizontalLine(x1, x2, y1 + dy);
let dy = dy - 1;
}
do Screen.drawHorizontalLine(x1, x2, y1);
return;
}
function void drawCircle(int x, int y, int radius) {
var int dx, dy, radiusPlusOne;
let dy = -radius;
let radiusPlusOne = radius + 1;
while (dy < radiusPlusOne) {
let dx = Math.sqrt((radius * radius) - (dy * dy));
do Screen.drawHorizontalLine(x - dx, x + dx, y + dy);
let dy = dy + 1;
}
return;
}
// LOCAL FUNCTIONS
function void drawHorizontalLine(int x1, int x2, int y) {
var int temp, leftAddress, rightAddress, yCell, leftEndOfLine, rightEndOfLine;
if (x1 > x2) {
let temp = x1;
let x1 = x2;
let x2 = temp;
}
let yCell = (y * 32);
let leftAddress = yCell + (x1 / 16);
let rightAddress = yCell + (x2 / 16);
let leftEndOfLine = ~(powers_of_two[x1 & 15] - 1);
let rightEndOfLine = powers_of_two[x2 & 15] - 1;
if (leftAddress = rightAddress) {
if (color) {let screenMem[leftAddress] = screenMem[leftAddress] | (leftEndOfLine & rightEndOfLine);}
else {let screenMem[leftAddress] = screenMem[leftAddress] & ~(leftEndOfLine & rightEndOfLine);}
}
else {
if (color) {
let screenMem[leftAddress] = screenMem[leftAddress] | leftEndOfLine;
let screenMem[rightAddress] = screenMem[rightAddress] | rightEndOfLine;
}
else {
let screenMem[leftAddress] = screenMem[leftAddress] & ~leftEndOfLine;
let screenMem[rightAddress] = screenMem[rightAddress] & ~rightEndOfLine;
}
if ((rightAddress - leftAddress) > 1) {
let leftAddress = leftAddress + 1;
while (leftAddress < rightAddress) {
let screenMem[leftAddress] = color;
let leftAddress = leftAddress + 1;
}
}
}
return;
}
}
If you could help my figure this out it would be greatly appreciated. Thanks! :)