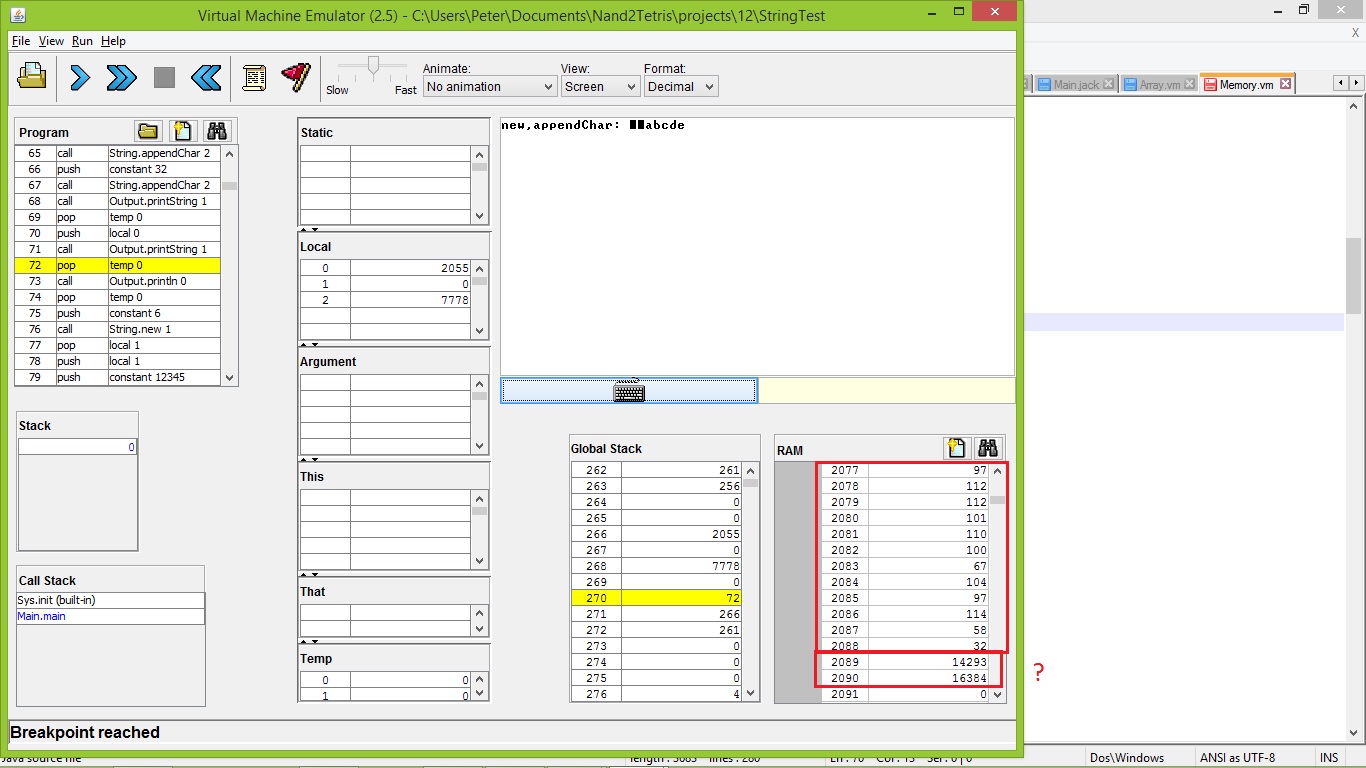
I'm stuck on a bug for my String class. I don't understand why it prints those last two black squares on the command:
do Output.printString("new,appendChar: ");
It seems to only happen when string literals are being printed as opposed to when printing string variables (hence "abcde" is printing out fine since it was stored in a variable and printed). If you look at the RAM section in the image, the first red square indicates the values of the character in the above string literal being stored in memory, which looks fine. I think the issue may be coming from the next two values 14293 and 16384. They show up whenever Memory.alloc is called for some reason and I don't know what their purpose is.
I have the following declared in my String.jack class for all String objects:
field Array str;
field int maxLen, len;
The way my appendChar() in the String class works is that it loops through the maxlength of the string and checks until str[i] == 0. I'm assuming that the end of a string is marked by the value 0 as a null character. Since "new,appendChar: " is 16 characters long exactly, there is no room for a 0 null character to mark the end of the string, which is why I think it continues reading the next RAM values 14293 and 16384. Any advice on my approach and how to fix this bug?
One more question, I'm unclear about the parameter of String.new(maxLength). The API says that it creates a string of at most maxLength characters. Does that include a null character? I get confused because in StringTest\Main.jack you have the following:
let s = String.new(6); // capacity 6, make sure length 5 will be printed
let s = s.appendChar(97);
let s = s.appendChar(98);
let s = s.appendChar(99);
let s = s.appendChar(100);
let s = s.appendChar(101);
Here maxLength is 6, but only 5 characters are being appended. I assumed the last one was for the 0 null character, and the comment seems to imply this as well. But why is it different for String literals such as in the command:
do Output.printString("new,appendChar: ");
in which only a space of 16 is allotted? Why do String Literals not have null characters in them while String objects do? Some clarification on the String.new(maxLength) method would be helpful.