The thing to understand in Figure 5.9 is that the "C" signals are a lot of individual signals. If you could magnify the figure you'd see the "C"s going into the top of the ALU as
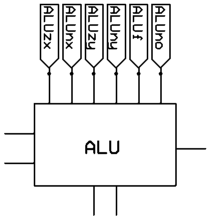
Magnifying the "C" outputs from the Decode block you'd see
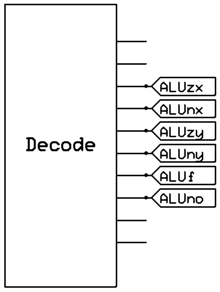
As Ybakos said, Decode is just a bunch of logic in the CPU. Here's a hint to get you started:
Some of the control signals must only be asserted (true) during A-instructions and some of them only during C-instructions. You will need a signal that indicates when an A-instruction is executing. It will also be handy to have a signal that indicates a C-instruction. You can generate these as
// Instruction decoder
Not(in=instruction[15], out=aInst);
Not(in=aInst, out=cInst);
When do you want to load the A register? For all A-instructions and for C-instructions with the d1 bit set. So you need a control signal to hook to the A-register's load:
loadAreg = aInst | (cInst & d1)
If you need more help, feel free to email me directly.
--Mark